Motorola SC140 User Manual
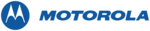
Setting Up the Stopwatch Timer Within an Application
7
Code 4. C Code for Clock-Cycle-to-Time Conversion
typedef enum { EONCE_SECOND, EONCE_MILLISECOND, EONCE_MICROSECOND } tunit;
unsigned long Convert_clock2time(unsigned long clock_ext, unsigned long clock_val,
short option)
{
unsigned long result;
switch(option)
{
case EONCE_SECOND:
result= clock_ext*MAX_32_BIT/CLOCK_SPEED + clock_val/CLOCK_SPEED;
break;
case EONCE_MILLISECOND:
result= clock_ext*MAX_32_BIT/(CLOCK_SPEED/1000)
+ clock_val/(CLOCK_SPEED/1000);
break;
case EONCE_MICROSECOND:
result= clock_ext*MAX_32_BIT/(CLOCK_SPEED/1000000)
+ clock_val/(CLOCK_SPEED/1000000);
break;
default: result=0; /* error condition */
break;
}
return result;
}
short option)
{
unsigned long result;
switch(option)
{
case EONCE_SECOND:
result= clock_ext*MAX_32_BIT/CLOCK_SPEED + clock_val/CLOCK_SPEED;
break;
case EONCE_MILLISECOND:
result= clock_ext*MAX_32_BIT/(CLOCK_SPEED/1000)
+ clock_val/(CLOCK_SPEED/1000);
break;
case EONCE_MICROSECOND:
result= clock_ext*MAX_32_BIT/(CLOCK_SPEED/1000000)
+ clock_val/(CLOCK_SPEED/1000000);
break;
default: result=0; /* error condition */
break;
}
return result;
}
3.5
Putting it All Together
Code 5 depicts the use of the stopwatch timer using the routines that have been described so far.
Code 5. Use of Stopwatch Timer Functions
long clock_ext,clock_val,clock_cycle,time_sec;
...
EOnCE_stopwatch_timer_init(); /* Execute once per program execution */
...
EOnCE_stopwatch_timer_start();
/*
* Code whose execution time is measured goes here
*/
EOnCE_stopwatch_timer_stop(&clock_ext, &clock_val);
/*
* Code whose execution time is measured goes here
*/
EOnCE_stopwatch_timer_stop(&clock_ext, &clock_val);
...
time_sec = Convert_clock2time(clock_ext, clock_val, EONCE_SECOND);
...
...
...
EOnCE_stopwatch_timer_start();
/*
* Code whose execution time is measured goes here
*/
EOnCE_stopwatch_timer_stop(&clock_ext, &clock_val);
/*
* Code whose execution time is measured goes here
*/
EOnCE_stopwatch_timer_stop(&clock_ext, &clock_val);
...
time_sec = Convert_clock2time(clock_ext, clock_val, EONCE_SECOND);
The calls to the stopwatch timer functions can be made from different locations in the application code, not
necessarily from within one subroutine. The
necessarily from within one subroutine. The
EOnCE_stopwatch_timer_start
() and
EOnCE_stopwatch_timer_stop
() can be called more than once to measure the time consumed in different
modules as desired.