Microchip Technology XC8 Standard Compiler (Workstation) SW006021-1 SW006021-1 User Manual
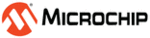
Product codes
SW006021-1
Library Functions
2012 Microchip Technology Inc.
DS52053B-page 363
See Also
strlen()
, strncmp(), strcpy(), strcat()
Return Value
A signed integer less than, equal to or greater than zero.
Note
Other C implementations may use a different collating sequence; the return value is
negative, zero, or positive; i.e., do not test explicitly for negative one (-1) or one (1).
negative, zero, or positive; i.e., do not test explicitly for negative one (-1) or one (1).
STRCPY
Synopsis
#include <string.h>
char * strcpy (char * s1, const char * s2)
Description
This function copies a null terminated string
s2
to a character array pointed to by
s1
.
The destination array must be large enough to hold the entire string, including the null
terminator.
terminator.
Example
#include <string.h>
#include <stdio.h>
void
main (void)
{
char buffer[256];
char * s1, * s2;
strcpy(buffer, "Start of line");
s1 = buffer;
s2 = "... end of line";
strcat(s1, s2);
printf("Length = %d\n", strlen(buffer));
printf("string = \"%s\"\n", buffer);
}
See Also
strncpy()
, strlen(), strcat(), strlen()
Return Value
The destination buffer
s1
is returned.
STRCSPN
Synopsis
#include <string.h>
size_t strcspn (const char * s1, const char * s2)
Description
The
strcspn()
function returns the length of the initial segment of the string pointed to
by
s1
which consists of characters NOT from the string pointed to by
s2
.