Microchip Technology XC8 Standard Compiler (Workstation) SW006021-1 SW006021-1 User Manual
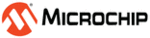
Product codes
SW006021-1
Library Functions
2012 Microchip Technology Inc.
DS52053B-page 375
Description
The function
utoa()
converts the unsigned contents of
val
into a string which is stored
into
buf
. The conversion is performed according to the radix specified in
base
.
buf
is
assumed to reference a buffer which has sufficient space allocated to it.
Example
#include <stdlib.h>
#include <stdio.h>
void
main (void)
{
char buf[10];
utoa(buf, 1234, 16);
printf("The buffer holds %s\n", buf);
}
See Also
strtol()
, itoa(), ltoa(), ultoa()
Return Value
This routine returns a copy of the buffer into which the result is written.
VA_START, VA_ARG, VA_END
Synopsis
#include <stdarg.h>
void va_start (va_list ap, parmN)
type va_arg (ap, type)
void va_end (va_list ap)
Description
These macros are provided to give access in a portable way to parameters to a function
represented in a prototype by the ellipsis symbol (...), where type and number of
arguments supplied to the function are not known at compile time.
represented in a prototype by the ellipsis symbol (...), where type and number of
arguments supplied to the function are not known at compile time.
The right most parameter to the function (shown as
parmN
) plays an important role in
these macros, as it is the starting point for access to further parameters. In a function
taking variable numbers of arguments, a variable of type
taking variable numbers of arguments, a variable of type
va_list
should be declared,
then the macro
va_start()
invoked with that variable and the name of
parmN
. This will
initialize the variable to allow subsequent calls of the macro
va_arg()
to access suc-
cessive parameters.
Each call to
va_arg()
requires two arguments; the variable previously defined and a
type name which is the type that the next parameter is expected to be. Note that any
arguments thus accessed will have been widened by the default conventions to int,
unsigned int
arguments thus accessed will have been widened by the default conventions to int,
unsigned int
or double. For example, if a character argument has been passed, it
should be accessed by
va_arg(ap, int)
since the char will have been widened to
int
.
An example is given below of a function taking one integer parameter, followed by a
number of other parameters. In this example the function expects the subsequent
parameters to be s to char, but note that the compiler is not aware of this, and it is the
programmers responsibility to ensure that correct arguments are supplied.
number of other parameters. In this example the function expects the subsequent
parameters to be s to char, but note that the compiler is not aware of this, and it is the
programmers responsibility to ensure that correct arguments are supplied.