Microchip Technology XC8 Standard Compiler (Workstation) SW006021-1 SW006021-1 User Manual
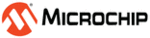
Product codes
SW006021-1
How To’s
2012 Microchip Technology Inc.
DS52053B-page 59
A stub for the putch function can be found in the compiler’s sources directory. Copy
it into your project then modify it to send the single byte parameter passed to it to the
required destination. Before you can use printf, peripherals that you use will need to
be initialized in the usual way. Here is an example of putch for a USART on a mid-range
device.
it into your project then modify it to send the single byte parameter passed to it to the
required destination. Before you can use printf, peripherals that you use will need to
be initialized in the usual way. Here is an example of putch for a USART on a mid-range
device.
void putch(char data) {
while( ! TXIF) // check buffer
continue; // wait till ready
TXREG = data; // send data
}
You can get printf to send to one of several destinations by using a global variable
to indicate your choice. Have the putch function send the byte to one of several des-
tinations based on the contents of this variable.
to indicate your choice. Have the putch function send the byte to one of several des-
tinations based on the contents of this variable.
3.5.8
How Do I Calibrate the Oscillator on My Device?
Some devices allow for calibration of their internal oscillators, see your device data
sheet. The runtime startup code generated by the compiler, see Section 5.10.1 “Run-
time Startup Code”, will by default provide code that performs oscillator calibration.
This can be disabled, if required, using an option, see Section 4.8.50 “--RUNTIME:
Specify Runtime Environment”.
sheet. The runtime startup code generated by the compiler, see Section 5.10.1 “Run-
time Startup Code”, will by default provide code that performs oscillator calibration.
This can be disabled, if required, using an option, see Section 4.8.50 “--RUNTIME:
Specify Runtime Environment”.
3.5.9
How Do I Place Variables in The PIC18’s External Program
Memory?
Memory?
If all you mean to do is place read-only variables in program memory, qualify them as
const
const
, see Section 5.5.3 “Variables in Program Space”. If you intend the variables
to be located in the external program memory then use the far qualifier and specify
the memory using the --RAM option, see Section 4.8.48 “--RAM: Adjust RAM
Ranges”. The compiler will allow far-qualified variables to be modified. Note that the
time to access these variables will be longer than for variables in the internal data mem-
ory. The access mode to external memory can be specified with an option, see
Section 4.8.26 “--EMI: Select External Memory Interface Operating Mode”.
the memory using the --RAM option, see Section 4.8.48 “--RAM: Adjust RAM
Ranges”. The compiler will allow far-qualified variables to be modified. Note that the
time to access these variables will be longer than for variables in the internal data mem-
ory. The access mode to external memory can be specified with an option, see
Section 4.8.26 “--EMI: Select External Memory Interface Operating Mode”.
3.5.10
How Can I Implement a Delay in My Code?
If an accurate delay is required, or if there are other tasks that can be performed during
the delay, then using a timer to generate an interrupt is the best way to proceed.
the delay, then using a timer to generate an interrupt is the best way to proceed.
If these are not issues in your code, then you can use the compiler’s in-built delay
pseudo-functions: _delay, __delay_ms or __delay_us, see Appendix A. “Library
Functions”. These all expand into in-line assembly instructions or a (nested) loop of
instructions which will consume the specified number of cycles or time. The delay argu-
ment must be a constant and less than approximately 179,200 for PIC18 devices and
approximately 50,659,000 for other devices.
pseudo-functions: _delay, __delay_ms or __delay_us, see Appendix A. “Library
Functions”. These all expand into in-line assembly instructions or a (nested) loop of
instructions which will consume the specified number of cycles or time. The delay argu-
ment must be a constant and less than approximately 179,200 for PIC18 devices and
approximately 50,659,000 for other devices.
Note that these code sequences will only use the NOP instruction and/or instructions
which form a loop. The alternate PIC18-only versions of these pseudo-functions, e.g.,
_delaywdt
which form a loop. The alternate PIC18-only versions of these pseudo-functions, e.g.,
_delaywdt
, may use the CLRWDT instruction as well. See also Appendix A. “Library
3.5.11
How Can I Rotate a Variable?
The C language does not have a rotate operator, but rotations can be performed using
the shift and bitwise OR operators. Since the PIC devices have a rotate instruction, the
compiler will look for code expressions that implement rotates (using shifts and ORs)
and use the rotate instruction in the generated output wherever possible, see
Section 5.6.2 “Rotation”.
the shift and bitwise OR operators. Since the PIC devices have a rotate instruction, the
compiler will look for code expressions that implement rotates (using shifts and ORs)
and use the rotate instruction in the generated output wherever possible, see
Section 5.6.2 “Rotation”.