Microchip Technology SW006023-1N Data Sheet
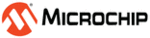
MPLAB
®
XC32 C/C++ Compiler User’s Guide
DS51686E-page 118
2012 Microchip Technology Inc.
Another problem that frequently occurs is with the bitwise compliment operator, ~. This
operator toggles each bit within a value. Consider the following code:
operator toggles each bit within a value. Consider the following code:
unsigned char count, c;
c = 0x55;
if( ~c == 0xAA)
count++;
If c contains the value 0x55, it often assumed that ~c will produce 0xAA, however the
result is 0xFFFFFFAA and so the comparison in the above example would fail. The
compiler may be able to issue a mismatched comparison error to this effect in some
circumstances. Again, a cast could be used to change this behavior.
result is 0xFFFFFFAA and so the comparison in the above example would fail. The
compiler may be able to issue a mismatched comparison error to this effect in some
circumstances. Again, a cast could be used to change this behavior.
The consequence of integral promotion as illustrated above is that operations are not
performed with char -type operands, but with int -type operands. However there are
circumstances when the result of an operation is identical regardless of whether the
operands are of type char or int. In these cases, the MPLAB XC32 C/C++ Compiler
will not perform the integral promotion so as to increase the code efficiency. Consider
the following example:
performed with char -type operands, but with int -type operands. However there are
circumstances when the result of an operation is identical regardless of whether the
operands are of type char or int. In these cases, the MPLAB XC32 C/C++ Compiler
will not perform the integral promotion so as to increase the code efficiency. Consider
the following example:
unsigned char a, b, c;
a = b + c;
Strictly speaking, this statement requires that the values of b and c should be promoted
to unsigned int, the addition performed, the result of the addition cast to the type of
a
to unsigned int, the addition performed, the result of the addition cast to the type of
a
, and then the assignment can take place. Even if the result of the unsigned int
addition of the promoted values of b and c was different to the result of the unsigned
char
char
addition of these values without promotion, after the unsigned int result was
converted back to unsigned char, the final result would be the same. If an 8-bit addi-
tion is more efficient than a 32-bit addition, the compiler will encode the former.
tion is more efficient than a 32-bit addition, the compiler will encode the former.
If, in the above example, the type of a was unsigned int, then integral promotion
would have to be performed to comply with the ANSI C standard.
would have to be performed to comply with the ANSI C standard.
8.3
TYPE REFERENCES
Another way to refer to the type of an expression is with the typeof keyword. This is
a non-standard extension to the language. Using this feature reduces your code
portability.
a non-standard extension to the language. Using this feature reduces your code
portability.
The syntax for using this keyword looks like sizeof, but the construct acts
semantically like a type name defined with typedef.
semantically like a type name defined with typedef.
There are two ways of writing the argument to typeof: with an expression or with a
type. Here is an example with an expression:
type. Here is an example with an expression:
typeof (x[0](1))
This assumes that x is an array of functions; the type described is that of the values of
the functions.
the functions.
Here is an example with a typename as the argument:
typeof (int *)
Here the type described is a pointer to int.
If you are writing a header file that must work when included in ANSI C programs, write
_ _typeof__
_ _typeof__
instead of typeof.
A typeof construct can be used anywhere a typedef name could be used. For
example, you can use it in a declaration, in a cast, or inside of sizeof or typeof.
example, you can use it in a declaration, in a cast, or inside of sizeof or typeof.
• This declares y with the type of what x points to:
typeof (*x) y;
• This declares y as an array of such values:
typeof (*x) y[4];