Microchip Technology SW006023-1N Data Sheet
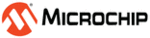
MPLAB
®
XC32 C/C++ Compiler User’s Guide
DS51686E-page 98
2012 Microchip Technology Inc.
6.6
STRUCTURES AND UNIONS
MPLAB XC32 C/C++ Compiler supports struct and union types. Structures and
unions only differ in the memory offset applied to each member.
unions only differ in the memory offset applied to each member.
These types will be at least 1 byte wide. Bit fields are fully supported.
No padding of structure members is added.
Structures and unions may be passed freely as function arguments and function return
values. Pointers to structures and unions are fully supported.
values. Pointers to structures and unions are fully supported.
6.6.1
Structure and Union Qualifiers
The MPLAB XC32 C/C++ Compiler supports the use of type qualifiers on structures.
When a qualifier is applied to a structure, all of its members will inherit this qualification.
In the following example the structure is qualified const.
When a qualifier is applied to a structure, all of its members will inherit this qualification.
In the following example the structure is qualified const.
const struct {
int number;
int *ptr;
} record = { 0x55, &i };
In this case, the entire structure will be placed into the program memory and each
member will be read-only. Remember that all members are usually initialized if a struc-
ture is const as they cannot be initialized at runtime.
member will be read-only. Remember that all members are usually initialized if a struc-
ture is const as they cannot be initialized at runtime.
If the members of the structure were individually qualified const, but the structure was
not, then the structure would be positioned into RAM, but each member would be
read-only. Compare the following structure with the above.
not, then the structure would be positioned into RAM, but each member would be
read-only. Compare the following structure with the above.
struct {
const int number;
int * const ptr;
} record = { 0x55, &i};
6.6.2
Bit Fields in Structures
MPLAB XC32 C/C++ Compiler fully supports bit fields in structures.
Bit fields are always allocated within 8-bit storage units, even though it is usual to use
the type unsigned int in the definition. Storage units are aligned on a 32-bit bound-
ary, although this can be changed using the packed attribute.
the type unsigned int in the definition. Storage units are aligned on a 32-bit bound-
ary, although this can be changed using the packed attribute.
The first bit defined will be the Least Significant bit of the word in which it will be stored.
When a bit field is declared, it is allocated within the current 8-bit unit if it will fit; other-
wise, a new byte is allocated within the structure. Bit fields can never cross the bound-
ary between 8-bit allocation units. For example, the declaration:
When a bit field is declared, it is allocated within the current 8-bit unit if it will fit; other-
wise, a new byte is allocated within the structure. Bit fields can never cross the bound-
ary between 8-bit allocation units. For example, the declaration:
struct {
unsigned lo : 1;
unsigned dummy : 6;
unsigned hi : 1;
} foo;
will produce a structure occupying 1 byte. If foo was ultimately linked at address 10H,
the field lo will be bit 0 of address 10H; hi will be bit 7 of address 10H. The Least Sig-
nificant bit of dummy will be bit 1 of address 10H and the Most Significant bit of dummy
will be bit 6 of address 10h.
the field lo will be bit 0 of address 10H; hi will be bit 7 of address 10H. The Least Sig-
nificant bit of dummy will be bit 1 of address 10H and the Most Significant bit of dummy
will be bit 6 of address 10h.