Microchip Technology DM164130-9 User Manual
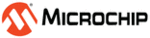
PICkit™ 3 Starter Kit User’s Guide
DS41628B-page 62
2012 Microchip Technology Inc.
EXAMPLE 3-25:
If Delay2 is ‘0’, then the return instruction will not be executed, and instead skipped.
3.6.5.2.2
rcall
A relative call should be used if the location to jump to is within 1K of the current
location of the Program Counter. The reason is that rcall consumes only one word
location of the Program Counter. The reason is that rcall consumes only one word
of program spaces, whereas a regular call takes two words.
3.6.6
Assembly
3.6.6.1
BOTH
EXAMPLE 3-26:
The main loop is now more readable than before. There are separate modules, or func-
tions for the ADC, delay loop, and rotate. Be sure to return after a call, and not a
tions for the ADC, delay loop, and rotate. Be sure to return after a call, and not a
or goto.
The CheckIfZero is necessary so that the delay loop does not rollover to 255 from 0.
The CheckIfZero is necessary so that the delay loop does not rollover to 255 from 0.
If this call is omitted and the ADC result is ‘0’, then the LEDs will rotate very slowly.
3.6.7
C Language
This implementation is much easier to understand.
EXAMPLE 3-27:
The routine will delay at least 50 ms when the ADC result is zero. For each increment
of the returned ADC value, the loop will pause for 2 ms.
This lesson also introduces function calls.
of the returned ADC value, the loop will pause for 2 ms.
This lesson also introduces function calls.
This is the equivalent of implementing a call in assembly. The program counter will
go to where this ADC function is in program space and execute code. It will then return
a single value and assign it to delay.
A key note is that any function that is instantiated after the main function must have a
a single value and assign it to delay.
A key note is that any function that is instantiated after the main function must have a
prototype.
tstfsz Delay2 ;if the ADC result is NOT '0', then simply return to MainLoop
return ;return to MainLoop
return ;return to MainLoop
MainLoop:
call A2d ;get the ADC result
;top 8 MSbs are now in the working register (Wreg)
movwf Delay2 ;move ADC result into the outer delay loop
call CheckIfZero ;if ADC result is zero, load in a value of '1' or else
the delay loop will decrement starting at 255
call DelayLoop ;delay the next LED from turning ON
call Rotate ;rotate the LEDs
call A2d ;get the ADC result
;top 8 MSbs are now in the working register (Wreg)
movwf Delay2 ;move ADC result into the outer delay loop
call CheckIfZero ;if ADC result is zero, load in a value of '1' or else
the delay loop will decrement starting at 255
call DelayLoop ;delay the next LED from turning ON
call Rotate ;rotate the LEDs
bra MainLoop ;do this forever
__delay_ms(50); //delay for AT LEAST 50ms
while (delay-- != 0) __delay_ms(2); //decrement the 8 MSbs of the ADC and delay
2ms for each
while (delay-- != 0) __delay_ms(2); //decrement the 8 MSbs of the ADC and delay
2ms for each
delay = adc(); //grab the top 8 MSbs
unsigned char adc(void); //prototype