Microchip Technology SW006022-1N Data Sheet
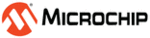
MPLAB
®
XC16 C Compiler User’s Guide
DS52071B-page 120
2012 Microchip Technology Inc.
A code example for this would be:
file1.c
/* to be compiled -mlarge-arrays */
__prog__ int array1[48000] __attribute__((space(prog)));
__prog__ int array2[48000] __attribute__((space(prog)));
int access_large_array(__prog__ int *array, unsigned long index) {
return array[index];
}
file2.c
/* to be compiled without -mlarge-arrays */
extern __prog__ int array1[] __attribute__((space(prog)));
extern __prog__ int array2[] __attribute__((space(prog)));
extern int access_large_array(__prog__ int *array,
unsigned long index);
main() {
fprintf(stderr,"Answer is: %d\n", access_large_array(array1,
39543));
fprintf(stderr,"Answer is: %d\n", access_large_array(array2,
16));
}
7.3.1.4
CHANGING NON-AUTO VARIABLE ALLOCATION
As described in Section 7.2 “Address Spaces”, the compiler arranges for data to be
placed into sections, depending on the memory model used and whether or not the
data is initialized. When modules are combined at link time, the linker determines the
starting addresses of the various sections based on their attributes.
placed into sections, depending on the memory model used and whether or not the
data is initialized. When modules are combined at link time, the linker determines the
starting addresses of the various sections based on their attributes.
Cases may arise when a specific variable must be located at a specific address, or
within some range of addresses. The easiest way to accomplish this is by using the
address
within some range of addresses. The easiest way to accomplish this is by using the
address
attribute, described in Chapter 5. “Differences Between MPLAB XC16
and ANSI C”. For example, to locate variable Mabonga at address 0x1000 in data
memory:
memory:
int
__
attribute
_ _
((address(0x1000))) Mabonga = 1;
A group of common variables may be allocated into a named section, complete with
address specifications:
address specifications:
int
__
attribute
_ _
((section("mysection"), address(0x1234))), foo;
7.3.1.5
DATA MEMORY ALLOCATION MACROS
Macros that may be used to allocate space in data memory are discussed below. There
are two types: those that require an argument and those that do not.
are two types: those that require an argument and those that do not.
The following macros require an argument N that specifies alignment. N must be a
power of two, with a minimum value of 2.
power of two, with a minimum value of 2.
#define _XBSS(N)
_ _
attribute
_ _
((space(xmemory), aligned(N)))
#define _XDATA(N)
_ _
attribute
_ _
((space(xmemory), aligned(N)))
#define _YBSS(N)
_ _
attribute
_ _
((space(ymemory), aligned(N)))
#define _YDATA(N)
_ _
attribute
_ _
((space(ymemory), aligned(N)))
#define _EEDATA(N)
_ _
attribute
_ _
((space(eedata), aligned(N)))
For example, to declare an uninitialized array in X memory that is aligned to a 32-byte
address:
address:
int _XBSS(32) xbuf[16];