Microchip Technology SW006023-2N Data Sheet
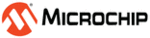
MPLAB
®
XC32 C/C++ Compiler User’s Guide
DS51686E-page 130
2012 Microchip Technology Inc.
10.7
FUNCTION RETURN VALUES
Function return values are returned in registers.
If a function needs to return an actual structure or union — not a pointer to such an
object — the called function copies this object to an area of memory that is reserved by
the caller. The caller passes the address of this memory area in register $4 when the
function is called. The function also returns a pointer to the returned object in register
v0
object — the called function copies this object to an area of memory that is reserved by
the caller. The caller passes the address of this memory area in register $4 when the
function is called. The function also returns a pointer to the returned object in register
v0
. Having the caller supply the return object’s space allows re-entrance.
10.8
CALLING FUNCTIONS
By default, functions are called using the direct form of the call (jal) instruction. This
allows calls to destinations within a 256 MB segment. This operation can be changed
through the use of attributes applied to functions or command-line options so that a lon-
ger, but unrestricted, call is made.
allows calls to destinations within a 256 MB segment. This operation can be changed
through the use of attributes applied to functions or command-line options so that a lon-
ger, but unrestricted, call is made.
The -mlong-calls option, see Section 3.9.1 “Options Specific to PIC32MX
Devices”, forces a register form of the call to be employed by default. Generated code
is longer, but calls are not limited in terms of the destination address.
Devices”, forces a register form of the call to be employed by default. Generated code
is longer, but calls are not limited in terms of the destination address.
The attributes longcall or far can be used with a function definition to always
enforce the longer call sequence for that function. The near attribute can be used with
a function so that calls to it use the shorter direct call, even if the -mlong-calls option
is in force.
enforce the longer call sequence for that function. The near attribute can be used with
a function so that calls to it use the shorter direct call, even if the -mlong-calls option
is in force.
10.9
INLINE FUNCTIONS
By declaring a function inline, you can direct the compiler to integrate that function’s
code into the code for its callers. This usually makes execution faster by eliminating the
function-call overhead. In addition, if any of the actual argument values are constant,
their known values may permit simplifications at compile time, so that not all of the
inline function’s code needs to be included. The effect on code size is less predictable.
Machine code may be larger or smaller with inline functions, depending on the
particular case.
code into the code for its callers. This usually makes execution faster by eliminating the
function-call overhead. In addition, if any of the actual argument values are constant,
their known values may permit simplifications at compile time, so that not all of the
inline function’s code needs to be included. The effect on code size is less predictable.
Machine code may be larger or smaller with inline functions, depending on the
particular case.
To declare a function inline, use the inline keyword in its declaration, like this:
inline int
inc (int *a)
{
(*a)++;
}
(If you are using the -traditional option or the -ansi option, write __inline__
instead of inline.) You can also make all “simple enough” functions inline with the
command-line option -finline-functions. The compiler heuristically decides
which functions are simple enough to be worth integrating in this way, based on an
estimate of the function’s size.
instead of inline.) You can also make all “simple enough” functions inline with the
command-line option -finline-functions. The compiler heuristically decides
which functions are simple enough to be worth integrating in this way, based on an
estimate of the function’s size.
Note:
Function inlining will only take place when the function’s definition is visible
(not just the prototype). In order to have a function inlined into more than
one source file, the function definition may be placed into a header file that
is included by each of the source files.
(not just the prototype). In order to have a function inlined into more than
one source file, the function definition may be placed into a header file that
is included by each of the source files.
Note:
The inline keyword will only be recognized with -finline or
optimizations enabled.
optimizations enabled.