Microchip Technology SW006023-2N Data Sheet
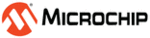
MPLAB
®
XC32 C/C++ Compiler User’s Guide
DS51686E-page 54
2012 Microchip Technology Inc.
3.4.2
Compiling Multiple C and C++ files
This section demonstrates how to compile and link multiple C and C++ files in a single
step.
step.
File 1
/* main.cpp */
#include <xc.h> // __XC_UART
#include <plib.h> // SYSTEMConfigPerformance()
#include <iostream>
using namespace std;
// Device-Specific Configuration-bit settings
#pragma config FPLLMUL=MUL_20, FPLLIDIV=DIV_2, FPLLODIV=DIV_1,
FWDTEN=OFF
#pragma config POSCMOD=HS, FNOSC=PRIPLL, FPBDIV=DIV_8
// add() must have C linkage
extern "C" {
extern unsigned int add(unsigned int a, unsigned int b);
}
int main(void) {
int myvalue = 6;
// Configure the target for max performance at 80 MHz.
SYSTEMConfigPerformance (80000000UL);
// Direct stdout to UART 1 for use with the simulator
__XC_UART = 1;
std::cout << "original value: " << myvalue << endl;
myvalue = add (myvalue, 3);
std::cout << "new value: " << myvalue << endl;
while(1);
}
File 2
/* ex3.c */
unsigned int
add(unsigned int a, unsigned int b)
{
return(a+b);
}
Compile both files by typing the following at the prompt:
xc32-g++ -mprocessor=32MX795F512L -o ex3.elf main.cpp ex3.c
The command compiles the modules main.cpp and ex3.c. The compiled modules
are linked with the compiler libraries for C++ and the executable file ex3.elf is cre-
ated.
are linked with the compiler libraries for C++ and the executable file ex3.elf is cre-
ated.
Note:
Use the xc32-g++ driver (as opposed to the xc32-gcc driver) in order to link
the project with the C++ support libraries necessary for the C++ source file
in the project.
the project with the C++ support libraries necessary for the C++ source file
in the project.