Microchip Technology SW006023-2N Data Sheet
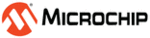
MPLAB
®
XC32 C/C++ Compiler User’s Guide
DS51686E-page 88
2012 Microchip Technology Inc.
4.5
USING SFRS FROM C CODE
The Special Function Registers (SFRs) are registers which control aspects of the MCU
operation or that of peripheral modules on the device. These registers are memory
mapped, which means that they appear at specific addresses in the device memory
map. With some registers, the bits within the register control independent features.
operation or that of peripheral modules on the device. These registers are memory
mapped, which means that they appear at specific addresses in the device memory
map. With some registers, the bits within the register control independent features.
Memory-mapped SFRs are accessed by special C variables that are placed at the
addresses of the registers and use special attributes. These variables can be accessed
like any ordinary C variable so that no special syntax is required to access SFRs.
addresses of the registers and use special attributes. These variables can be accessed
like any ordinary C variable so that no special syntax is required to access SFRs.
The SFR variables are predefined in header files and will be accessible once the
<xc.h>
<xc.h>
header file (see Section 4.3 “Device Header Files”) has been included into
your source code. Structures are also defined by these header files to allow access to
bits within the SFR.
bits within the SFR.
The names given to the C variables, which map over the registers and bit variables, or
bit fields, within the registers are based on the names specified in the device data
sheet. The names of the structures that hold the bit fields will typically be those of the
corresponding register followed by bits. For example, the following shows code that
includes the generic header file, clears PORTB as a whole and sets bit 2 of PORTB
using the structure/bit field definitions.
bit fields, within the registers are based on the names specified in the device data
sheet. The names of the structures that hold the bit fields will typically be those of the
corresponding register followed by bits. For example, the following shows code that
includes the generic header file, clears PORTB as a whole and sets bit 2 of PORTB
using the structure/bit field definitions.
#include <xc.h>
int main(void)
{
PORTB = 0x00;
PORTBbits.RB2 = 1;
}
For use with assembly, the PORTB register is declared as: .extern PORTB.
To confirm the names that are relevant for the device you are using, check the device
specific header file that <xc.h> will include for the definitions of each variable. These
files will be located in the pic32mx/include/proc directory of the compiler and will
have a name that represents the device. There is a one-to-one correlation between
device and header file name that will be included by <xc.h>, e.g. when compiling for
a PIC32MX360F512L device, the <xc.h> header file will include
<p32mx360f512l.h>
specific header file that <xc.h> will include for the definitions of each variable. These
files will be located in the pic32mx/include/proc directory of the compiler and will
have a name that represents the device. There is a one-to-one correlation between
device and header file name that will be included by <xc.h>, e.g. when compiling for
a PIC32MX360F512L device, the <xc.h> header file will include
<p32mx360f512l.h>
. Remember that you do not need to include this chip-specific
file into your source code; it is automatically included by <xc.h>.
Some of the PIC32 SFRs have associated registers that allow the bits within the SFR
to be set, cleared or toggled atomically. For example, the PORTB SFR has the write-only
registers PORTBSET, PORTBCLR and PORTBINV associated with it. Writing a ‘1’ to a bit
location in these registers sets, clears or toggles, respectively, the corresponding bit in
the PORTB SFR. So to set bit 1 in PORTB, you can use the following code:
to be set, cleared or toggled atomically. For example, the PORTB SFR has the write-only
registers PORTBSET, PORTBCLR and PORTBINV associated with it. Writing a ‘1’ to a bit
location in these registers sets, clears or toggles, respectively, the corresponding bit in
the PORTB SFR. So to set bit 1 in PORTB, you can use the following code:
PORTBSET = 0x2;
or alternatively, using macros provided in the device header files:
PORTBSET = _PORTB_RB1_MASK;
The same operation can also be achieved using the peripheral library functions, for
example
example
mPORTBSetBits(BIT_1);
Always ensure that you confirm the operation of peripheral modules from the device
data sheet.
data sheet.
Note:
The symbols PORTB and PORTBbits refer to the same register and resolve
to the same address. Writing to one register will change the values held by
both.
to the same address. Writing to one register will change the values held by
both.