Microchip Technology SW006022-2N Data Sheet
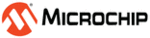
MPLAB
®
XC16 C Compiler User’s Guide
DS52071B-page 158
2012 Microchip Technology Inc.
10.6
INLINE FUNCTIONS
By declaring a function inline, you can direct the compiler to integrate that function’s
code into the code for its callers. This usually makes execution faster by eliminating the
function-call overhead. In addition, if any of the actual argument values are constant,
their known values may permit simplifications at compile time, so that not all of the
inline function’s code needs to be included. The effect on code size is less predictable.
Machine code may be larger or smaller with inline functions, depending on the
particular case.
code into the code for its callers. This usually makes execution faster by eliminating the
function-call overhead. In addition, if any of the actual argument values are constant,
their known values may permit simplifications at compile time, so that not all of the
inline function’s code needs to be included. The effect on code size is less predictable.
Machine code may be larger or smaller with inline functions, depending on the
particular case.
To declare a function inline, use the inline keyword in its declaration, like this:
inline int
inc (int *a)
{
(*a)++;
}
(If you are using the -traditional option or the -ansi option, write __inline__
instead of inline.) You can also make all “simple enough” functions inline with the
command-line option -finline-functions. The compiler heuristically decides
which functions are simple enough to be worth integrating in this way, based on an
estimate of the function’s size.
instead of inline.) You can also make all “simple enough” functions inline with the
command-line option -finline-functions. The compiler heuristically decides
which functions are simple enough to be worth integrating in this way, based on an
estimate of the function’s size.
Certain usages in a function definition can make it unsuitable for inline substitution.
Among these usages are: use of varargs, use of alloca, use of variable-sized data,
use of computed goto and use of nonlocal goto. Using the command-line option
-Winline
Among these usages are: use of varargs, use of alloca, use of variable-sized data,
use of computed goto and use of nonlocal goto. Using the command-line option
-Winline
will warn when a function marked inline could not be substituted, and will
give the reason for the failure.
In compiler syntax, the inline keyword does not affect the linkage of the function.
When a function is both inline and static, if all calls to the function are integrated
into the caller and the function’s address is never used, then the function’s own
assembler code is never referenced. In this case, the compiler does not actually output
assembler code for the function, unless you specify the command-line option
-fkeep-inline-functions
into the caller and the function’s address is never used, then the function’s own
assembler code is never referenced. In this case, the compiler does not actually output
assembler code for the function, unless you specify the command-line option
-fkeep-inline-functions
. Some calls cannot be integrated for various reasons
(in particular, calls that precede the function’s definition cannot be integrated and
neither can recursive calls within the definition). If there is a nonintegrated call, then the
function is compiled to assembler code as usual. The function must also be compiled
as usual if the program refers to its address, because that can’t be inlined. The compiler
will only eliminate inline functions if they are declared to be static and if the function
definition precedes all uses of the function.
neither can recursive calls within the definition). If there is a nonintegrated call, then the
function is compiled to assembler code as usual. The function must also be compiled
as usual if the program refers to its address, because that can’t be inlined. The compiler
will only eliminate inline functions if they are declared to be static and if the function
definition precedes all uses of the function.
When an inline function is not static, then the compiler must assume that there
may be calls from other source files. Since a global symbol can be defined only once
in any program, the function must not be defined in the other source files, so the calls
therein cannot be integrated. Therefore, a non-static inline function is always
compiled on its own in the usual fashion.
may be calls from other source files. Since a global symbol can be defined only once
in any program, the function must not be defined in the other source files, so the calls
therein cannot be integrated. Therefore, a non-static inline function is always
compiled on its own in the usual fashion.
Note:
Function inlining will only take place when the function’s definition is visible
at the call site (not just the prototype). In order to have a function inlined into
more than one source file, the function definition may be placed into a
header file that is included by each of the source files.
at the call site (not just the prototype). In order to have a function inlined into
more than one source file, the function definition may be placed into a
header file that is included by each of the source files.
Note:
The inline keyword will only be recognized with -finline or
optimizations enabled.
optimizations enabled.