Microchip Technology SW006022-2N Data Sheet
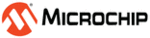
MPLAB
®
XC16 C COMPILER
USER’S GUIDE
2012 Microchip Technology Inc.
DS52071B-page 189
Chapter 13. Mixing C and Assembly Code
13.1
INTRODUCTION
This section describes how to use assembly language and C modules together. It gives
examples of using C variables and functions in assembly code and examples of using
assembly language variables and functions in C.
examples of using C variables and functions in assembly code and examples of using
assembly language variables and functions in C.
Items discussed are:
assembly language modules may be assembled, then linked with compiled C
modules.
modules.
• Using Inline Assembly Language – assembly language instructions may be
embedded directly into the C code. The inline assembler supports both simple
(non-parameterized) assembly language statement, as well as extended
(parameterized) statements (where C variables can be accessed as operands of
an assembler instruction).
(non-parameterized) assembly language statement, as well as extended
(parameterized) statements (where C variables can be accessed as operands of
an assembler instruction).
• Predefined Assembly Macros – a list of predefined assembly-code macros to be
used in C code is provided.
13.2
MIXING ASSEMBLY LANGUAGE AND C VARIABLES AND FUNCTIONS
The following guidelines indicate how to interface separate assembly language
modules with C modules.
modules with C modules.
• Follow the register conventions described in 9.2 “Register Variables”. In particu-
lar, registers W0-W7 are used for parameter passing. An assembly language
function will receive parameters, and should pass arguments to called functions,
in these registers.
function will receive parameters, and should pass arguments to called functions,
in these registers.
• Functions not called during interrupt handling must preserve registers W8-W15.
That is, the values in these registers must be saved before they are modified and
restored before returning to the calling function. Registers W0-W7 may be used
without restoring their values.
restored before returning to the calling function. Registers W0-W7 may be used
without restoring their values.
• Interrupt functions must preserve all registers. Unlike a normal function call, an
interrupt may occur at any point during the execution of a program. When return-
ing to the normal program, all registers must be as they were before the interrupt
occurred.
ing to the normal program, all registers must be as they were before the interrupt
occurred.
• Variables or functions declared within a separate assembly file that will be
referenced by any C source file should be declared as global using the assembler
directive.global. External symbols should be preceded by at least one
underscore. The C function main is named _main in assembly and conversely an
assembly symbol _do_something will be referenced in C as do_something.
Undeclared symbols used in assembly files will be treated as externally defined.
directive.global. External symbols should be preceded by at least one
underscore. The C function main is named _main in assembly and conversely an
assembly symbol _do_something will be referenced in C as do_something.
Undeclared symbols used in assembly files will be treated as externally defined.
The following example shows how to use variables and functions in both assembly
language and C regardless of where they were originally defined.
language and C regardless of where they were originally defined.
The file ex1.c defines foo and cVariable to be used in the assembly language file.
The C file also shows how to call an assembly function, asmFunction, and how to
access the assembly defined variable, asmVariable.
The C file also shows how to call an assembly function, asmFunction, and how to
access the assembly defined variable, asmVariable.