Microchip Technology SW006022-2N Data Sheet
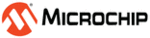
MPLAB
®
XC16 C Compiler User’s Guide
DS52071B-page 88
2012 Microchip Technology Inc.
EXAMPLE 4-1:
SAMPLE REAL-TIME CLOCK
/*
** Sample Real Time Clock for dsPIC
**
** Uses Timer1, TCY clock timer mode
** and interrupt on period match
*/
#include <xc.h>
/* Timer1 period for 1 ms with FOSC = 20 MHz */
#define TMR1_PERIOD 0x1388
struct clockType
{
unsigned int timer; /* countdown timer, milliseconds */
unsigned int ticks; /* absolute time, milliseconds */
unsigned int seconds; /* absolute time, seconds */
} volatile RTclock;
void reset_clock(void)
{
RTclock.timer = 0; /* clear software registers */
RTclock.ticks = 0;
RTclock.seconds = 0;
TMR1 = 0; /* clear timer1 register */
PR1 = TMR1_PERIOD; /* set period1 register */
T1CONbits.TCS = 0; /* set internal clock source */
IPC0bits.T1IP = 4; /* set priority level */
IFS0bits.T1IF = 0; /* clear interrupt flag */
IEC0bits.T1IE = 1; /* enable interrupts */
SRbits.IPL = 3; /* enable CPU priority levels 4-7*/
T1CONbits.TON = 1; /* start the timer*/
}
void
_ _
attribute
_ _
((
__
interrupt
_ _
,
_ _
auto_psv
_ _
)) _T1Interrupt(void)
{ static int sticks=0;
if (RTclock.timer > 0) /* if countdown timer is active */
RTclock.timer -= 1; /* decrement it */
RTclock.ticks++; /* increment ticks counter */
if (sticks++ > 1000)
{ /* if time to rollover */
sticks = 0; /* clear seconds ticks */
RTclock.seconds++; /* and increment seconds */
}
IFS0bits.T1IF = 0; /* clear interrupt flag */
return;
}