Atmel Evaluation Board using the SAM7SE Microcontroller AT91SAM7SE-EK AT91SAM7SE-EK Data Sheet
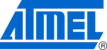
Product codes
AT91SAM7SE-EK
7
6295A–ATARM–27-Mar-07
Application Note
AT91C_BASE_WDTC->WDTC_WDMR = AT91C_WDTC_WDDIS;
3.2.1.8
Initializing Stacks
Each ARM mode has its own stack pointer (register sp); thus, each mode which is used in the
application must have its stack initialized.
application must have its stack initialized.
Since stacks are descending, i.e. the stack pointer decreases in value when data is stored, the
first stack pointer is located at the top of the internal SRAM. A particular length is reserved for
each mode, depending on its uses. Supervisor and user modes usually have big stacks, IRQ
and FIQ modes have a medium-sized stack, and other modes most often have only a few bytes.
In this example, only the Supervisor (SVC) and IRQ modes are used.
first stack pointer is located at the top of the internal SRAM. A particular length is reserved for
each mode, depending on its uses. Supervisor and user modes usually have big stacks, IRQ
and FIQ modes have a medium-sized stack, and other modes most often have only a few bytes.
In this example, only the Supervisor (SVC) and IRQ modes are used.
Stack initialization is done by entering each mode one after another, setting r13 to the correct
value. The top memory address is stored in a register, and decremented each time the stack
pointer is set. Note that interrupts are masked (i.e., I and F bits set) during this whole process,
except for the last mode (only F bit set). This results in the following code:
value. The top memory address is stored in a register, and decremented each time the stack
pointer is set. Note that interrupts are masked (i.e., I and F bits set) during this whole process,
except for the last mode (only F bit set). This results in the following code:
/*- Load top memory address in r0
ldr r0, =IRAMEND
/*- Enter Interrupt mode, setup stack */
msr CPSR_c, #ARM_MODE_IRQ | I_BIT | F_BIT
mov r13, r0
sub r0, r0, #IRQ_STACK_SIZE
/*- Enter Supervisor mode, setup stack, IRQs unmasked */
msr CPSR_c, #ARM_MODE_SVC | F_BIT
mov r13, r0
3.2.1.9
Initializing BSS and Data Segments
A binary file is usually divided into two segments: the first one holds the executable code of the
application, as well as read-only data (declared as const in C). The second segment contains
read/write data, i.e., data that can be modified. These two sections are called text and data,
respectively.
application, as well as read-only data (declared as const in C). The second segment contains
read/write data, i.e., data that can be modified. These two sections are called text and data,
respectively.
Variables in the data segment are said to be either uninitialized or initialized. In the first case, the
programmer has not set a particular value when declaring the variable; conversely, variables fall
in the second case when they have been declared with a value. Unitialized variables are held in
a special subsection called BSS (for Block Started by Symbol).
programmer has not set a particular value when declaring the variable; conversely, variables fall
in the second case when they have been declared with a value. Unitialized variables are held in
a special subsection called BSS (for Block Started by Symbol).
Whenever the application is loaded in the internal Flash memory of the chip, the Data segment
must be initialized at startup. This is necessary because read/write variables are located in
SRAM or SDRAM, not in Flash. Depending on the toolchain used, there might be library function
for doing this; for example, IAR Embedded Workbench
must be initialized at startup. This is necessary because read/write variables are located in
SRAM or SDRAM, not in Flash. Depending on the toolchain used, there might be library function
for doing this; for example, IAR Embedded Workbench
®
provides __segment_init().
Initialized data is contained in the binary file and loaded with the rest of the application in the
memory. Usually, it is located right after the text segment. This makes it easy to retrieve the
starting and ending address of the data to copy. To load these addresses faster, they are explic-
itly stored in the code using a compiler-specific instruction. Here is an example for the GNU
toolchain:
memory. Usually, it is located right after the text segment. This makes it easy to retrieve the
starting and ending address of the data to copy. To load these addresses faster, they are explic-
itly stored in the code using a compiler-specific instruction. Here is an example for the GNU
toolchain:
_lp_data:
.word _etext
.word _sdata
.word _edata