Atmel CAVR-4 Manual De Usuario
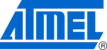
CAVR-4
126
Selecting data types and placing data in memory
AVR® IAR C/C++ Compiler
Reference Guide
Putting strings in flash
This can be done on individual strings or for the whole application/file using the option
--string_literals_in_flash
. Examples on how to put individual strings into
flash:
_ _flash char str1[] = "abcdef";
_ _flash char str2[] = "ghi";
_ _flash char _ _flash * pVar[] = { str1, str2 };
String literals cannot be put in flash automatically, but you can use a local static variable
instead:
instead:
#include <pgmspace.h>
void f (int i)
{
static _ _flash char sl[] = "%d cookies\n";
printf_P(sl, i);
}
This does not result in more code compared to allowing string literals in flash.
To use flash strings, you must use alternative library routines that expect flash strings.
A few such alternative functions are provided in the
A few such alternative functions are provided in the
pgmspace.h
header file. They are
flash alternatives for some common C library functions with an extension
_P
. For your
own code, you can always use the
_ _flash
keyword when passing the strings between
functions.
For reference information about the alternative functions, see AVR–specific library
functions, page 249.
functions, page 249.
USING EFFICIENT DATA TYPES
The data types you use should be considered carefully, because this can have a large
impact on code size and code speed.
impact on code size and code speed.
●
Use small and unsigned data types, (
unsigned char
and
unsigned short
)
unless your application really requires signed values.
●
Try to avoid 64-bit data types, such as
double
and
long long
.
●
Bitfields with sizes other than 1 bit should be avoided because they will result in
inefficient code compared to bit operations.
inefficient code compared to bit operations.
●
When using arrays, it is more efficient if the type of the index expression matches
the index type of the memory of the array. For
the index type of the memory of the array. For
_ _near
the index type is
int
.
●
Using floating-point types on a microprocessor without a math co-processor is very
inefficient, both in terms of code size and execution speed.
inefficient, both in terms of code size and execution speed.
●
Declaring a pointer to
const
data tells the calling function that the data pointed to
will not change, which opens for better optimizations.