Microchip Technology SW006022-1N Scheda Tecnica
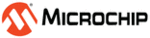
MPLAB
®
XC16 C Compiler User’s Guide
DS52071B-page 102
2012 Microchip Technology Inc.
Here is an example of code that may fail because the default type assigned to a literal
constant is not appropriate:
constant is not appropriate:
unsigned long int result;
unsigned char shifter;
void main(void)
{
shifter = 20;
result = 1 << shifter;
// code that uses result
}
The literal constant 1 will be assigned an int type; hence the result of the shift opera-
tion will be an int and the upper bits of the long variable, result, can never be set,
regardless of how much the literal constant is shifted. In this case, the value 1 shifted
left 20 bits will yield the result 0, not 0x100000.
tion will be an int and the upper bits of the long variable, result, can never be set,
regardless of how much the literal constant is shifted. In this case, the value 1 shifted
left 20 bits will yield the result 0, not 0x100000.
The following uses a suffix to change the type of the literal constant, hence ensure the
shift result has an unsigned long type.
shift result has an unsigned long type.
result = 1UL << shifter;
Floating-point literal constants have double type unless suffixed by f or F, in which
case it is a float constant. The suffixes l or L specify a long double type. In
MPLAB XC16, the double type equates to a 32-bit float type. The command line
option, -fno-short-double, may be use to specify double as a 64-bit long
double
case it is a float constant. The suffixes l or L specify a long double type. In
MPLAB XC16, the double type equates to a 32-bit float type. The command line
option, -fno-short-double, may be use to specify double as a 64-bit long
double
type.
Character literal constants are enclosed by single quote characters, ’, for example
’a’
’a’
. A character literal constant has int type, although this may be optimized to a
char
type later in the compilation.
Multi-byte character literal constants are supported by this implementation.
String constants, or string literals, are enclosed by double quote characters ", for exam-
ple "hello world". The type of string literal constants is const char * and the
character that make up the string may be stored in the program memory.
ple "hello world". The type of string literal constants is const char * and the
character that make up the string may be stored in the program memory.
To comply with the ANSI C standard, the compiler does not support the extended char-
acter set in characters or character arrays. Instead, they need to be escaped using the
backslash character, as in the following example:
acter set in characters or character arrays. Instead, they need to be escaped using the
backslash character, as in the following example:
const char name[] = "Bj\xf8k";
printf("%s's Resum\xe9", name); \\ prints "Bjørk's Resumé"
Defining and initializing a non-const array (i.e. not a pointer definition) with a string,
for example:
for example:
char ca[]= "two"; // "two" different to the above
is a special case and produces an array in data space which is initialized at startup with
the string "two", whereas a string literal constant used in other contexts represents an
unnamed array, accessed directly from its storage location.
the string "two", whereas a string literal constant used in other contexts represents an
unnamed array, accessed directly from its storage location.
The compiler will use the same storage location and label for strings that have identical
character sequences, except where the strings are used to initialize an array residing
in the data space as shown in the last statement in the previous example.
character sequences, except where the strings are used to initialize an array residing
in the data space as shown in the last statement in the previous example.
Two adjacent string literal constants (i.e. two strings separated only by white space) are
concatenated by the C preprocessor. Thus:
concatenated by the C preprocessor. Thus:
const char * cp = "hello " "world";
will assign the pointer with the address of the string "hello world ".