Microchip Technology SW006022-2N Scheda Tecnica
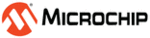
MPLAB
®
XC16 C Compiler User’s Guide
DS52071B-page 190
2012 Microchip Technology Inc.
Examples in this Section:
EXAMPLE 13-1:
MIXING C AND ASSEMBLY
/*
** file: ex1.c
*/
extern unsigned int asmVariable;
extern void asmFunction(void);
unsigned int cVariable;
void foo(void)
{
asmFunction();
asmVariable = 0x1234;
}
The file ex2.s defines asmFunction and asmVariable as required for use in a
linked application. The assembly file also shows how to call a C function, foo, and how
to access a C defined variable, cVariable.
linked application. The assembly file also shows how to call a C function, foo, and how
to access a C defined variable, cVariable.
;
; file: ex2.s
;
.text
.global _asmFunction
_asmFunction:
mov #0,w0
mov w0,_cVariable
return
.global _main
_main:
call _foo
return
.bss
.global _asmVariable
.align 2
_asmVariable: .space 2
.end
In the C file, ex1.c, external references to symbols declared in an assembly file are
declared using the standard extern keyword; note that asmFunction, or
_asmFunction
declared using the standard extern keyword; note that asmFunction, or
_asmFunction
in the assembly source, is a void function and is declared
accordingly.
In the assembly file, ex1.s, the symbols _asmFunction, _main and _asmVariable
are made globally visible through the use of the .global assembler directive and can
be accessed by any other source file. The symbol _main is only referenced and not
declared; therefore, the assembler takes this to be an external reference.
are made globally visible through the use of the .global assembler directive and can
be accessed by any other source file. The symbol _main is only referenced and not
declared; therefore, the assembler takes this to be an external reference.
The following compiler example shows how to call an assembly function with two
parameters. The C function main in call1.c calls the asmFunction in call2.s
with two parameters.
parameters. The C function main in call1.c calls the asmFunction in call2.s
with two parameters.