Microchip Technology SW006023-3N Data Sheet
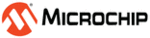
MPLAB
®
XC32 C/C++ Compiler User’s Guide
DS51686E-page 138
2012 Microchip Technology Inc.
EXAMPLE 11-8:
FULL TIMER 1 EXAMPLE WITH PERIPHERAL LIBRARY
/* Blink an LED on the PIC32MX Ethernet Starter Kit using the
* PIC32MX795F512L target device.
*/
#include <xc.h>
#include <plib.h>
#include <sys/attribs.h>
// Configuration Bit settings using the config pragma
// SYSCLK = 80 MHz (8MHz Crystal/ FPLLIDIV * FPLLMUL / FPLLODIV)
// PBCLK = 10 MHz
// Primary Osc w/PLL (XT+,HS+,EC+PLL)
// WDT OFF
// Other options are “do not care”
//
#pragma config FPLLMUL=MUL_20, FPLLIDIV=DIV_2, FPLLODIV=DIV_1, FWDTEN=OFF
#pragma config POSCMOD=HS, FNOSC=PRIPLL, FPBDIV=DIV_8
// Calculate the PR1 (period) at compile time
#define SYS_FREQ (80000000L)
#define PB_DIV 8
#define PRESCALE 256
#define TOGGLES_PER_SEC 1
#define T1_TICK (SYS_FREQ/PB_DIV/PRESCALE/TOGGLES_PER_SEC)
int main(void)
{
// STEP 1
// Configure the device for maximum performance but do not change the PBDIV
// Given the options, this function will change the flash wait states, RAM
// wait state and enable prefetch cache but will not change the PBDIV.
// The PBDIV value is already set via the config pragma FPBDIV option above.
SYSTEMConfig(SYS_FREQ, SYS_CFG_WAIT_STATES | SYS_CFG_PCACHE);
//~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// STEP 2. configure Timer 1 using internal clock, 1:256 prescale
OpenTimer1(T1_ON | T1_SOURCE_INT | T1_PS_1_256, T1_TICK);
// set up the timer interrupt with a priority of 7
ConfigIntTimer1(T1_INT_ON | T1_INT_PRIOR_7);
// enable multi-vector interrupts
INTEnableSystemMultiVectoredInt();
// configure PORTDbits.RD0 = output
mPORTDSetPinsDigitalOut(BIT_0);
while(1);
}
//~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// STEP 3. configure the Timer 1 interrupt handler
// Determine shadow-register or software-stack context saving at
// runtime by using the IPL7AUTO priority specifier.
// Note that the n value in IPLnAUTO _must_ match the priority
// of the timer1 interrupt source configured above.
#ifdef __cplusplus
// For C linkage when compiling for C++
extern "C" {
#endif /* __cplusplus */