Microchip Technology SW006023-2N Data Sheet
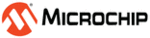
Supported Data Types and Variables
2012 Microchip Technology Inc.
DS51686E-page 103
unsigned char shifter;
int main(void)
{
shifter = 40;
result = 1 << shifter;
// code that uses result
}
The constant 1 will be assigned an int type hence the result of the shift operation will
be an int and the upper bits of the long variable, result, can never be set, regard-
less of how much the constant is shifted. In this case, the value 1 shifted left 40 bits will
yield the result 0, not 0x10000000000.
be an int and the upper bits of the long variable, result, can never be set, regard-
less of how much the constant is shifted. In this case, the value 1 shifted left 40 bits will
yield the result 0, not 0x10000000000.
The following uses a suffix to change the type of the constant, hence ensure the shift
result has an unsigned long type.
result has an unsigned long type.
result = 1UL << shifter;
Floating-point constants have double type unless suffixed by f or F, in which case it
is a float constant. The suffixes l or L specify a long double type.
is a float constant. The suffixes l or L specify a long double type.
Character constants are enclosed by single quote characters, ’, for example ’a’. A
character constant has int type, although this may be optimized to a char type later
in the compilation.
character constant has int type, although this may be optimized to a char type later
in the compilation.
Multi-byte character constants are accepted by the compiler but are not supported by
the standard libraries.
the standard libraries.
String constants, or string literals, are enclosed by double quote characters “, for exam-
ple “hello world”. The type of string constants is const char * and the character
that make up the string are stored in the program memory, as are all objects qualified
const
ple “hello world”. The type of string constants is const char * and the character
that make up the string are stored in the program memory, as are all objects qualified
const
.
To comply with the ANSI C standard, the compiler does not support the extended char-
acter set in characters or character arrays. Instead, they need to be escaped using the
backslash character, as in the following example:
acter set in characters or character arrays. Instead, they need to be escaped using the
backslash character, as in the following example:
const char name[] = "Bj\370rk";
printf(“%s's Resum\351”, name); \\ prints “Bjørk's Resumé”
Assigning a string literal to a pointer to a non-const char will generate a warning from
the compiler. This code is legal, but the behavior if the pointer attempts to write to the
string will fail. For example:
the compiler. This code is legal, but the behavior if the pointer attempts to write to the
string will fail. For example:
char * cp= “one”; // “one” in ROM, produces warning
const char * ccp= “two”; // “two” in ROM, correct
Defining and initializing a non-const array (i.e. not a pointer definition) with a string,
for example:
for example:
char ca[]= “two”; // “two” different to the above
is a special case and produces an array in data space which is initialized at start-up
with the string “two” (copied from program space), whereas a string constant used in
other contexts represents an unnamed const -qualified array, accessed directly in pro-
gram space.
with the string “two” (copied from program space), whereas a string constant used in
other contexts represents an unnamed const -qualified array, accessed directly in pro-
gram space.
The MPLAB XC32 C/C++ Compiler will use the same storage location and label for
strings that have identical character sequences. For example, in the code snippet
strings that have identical character sequences. For example, in the code snippet
if(strncmp(scp, “hello world”, 6) == 0)
fred = 0;
if(strcmp(scp, “hello world”) == 0)
fred++;