Microchip Technology SW006023-2N Data Sheet
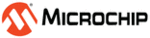
MPLAB
®
XC32 C/C++ Compiler User’s Guide
DS51686E-page 104
2012 Microchip Technology Inc.
the two identical character string greetings will share the same memory locations. The
link-time optimization must be enabled to allow this optimization when the strings may
be located in different modules.
link-time optimization must be enabled to allow this optimization when the strings may
be located in different modules.
Two adjacent string constants (i.e. two strings separated only by white space) are con-
catenated by the compiler. Thus:
catenated by the compiler. Thus:
const char * cp = “hello” “ world”;
will assign the pointer with the address of the string “hello world “.
6.10
STANDARD TYPE QUALIFIERS
Type qualifiers provide additional information regarding how an object may be used.
The MPLAB XC32 C/C++ Compiler supports both ANSI C qualifiers and additional spe-
cial qualifiers which are useful for embedded applications and which take advantage of
the PIC MCU architecture.
The MPLAB XC32 C/C++ Compiler supports both ANSI C qualifiers and additional spe-
cial qualifiers which are useful for embedded applications and which take advantage of
the PIC MCU architecture.
6.10.1
Const Type Qualifier
The MPLAB XC32 C/C++ Compiler supports the use of the ANSI type qualifiers const
and volatile.
and volatile.
The const type qualifier is used to tell the compiler that an object is read only and will
not be modified. If any attempt is made to modify an object declared const, the com-
piler will issue a warning or error.
not be modified. If any attempt is made to modify an object declared const, the com-
piler will issue a warning or error.
Usually a const object must be initialized when it is declared, as it cannot be assigned
a value at any point at runtime. For example:
a value at any point at runtime. For example:
const int version = 3;
will define version as being an int variable that will be placed in the program mem-
ory, will always contain the value 3, and which can never be modified by the program.
ory, will always contain the value 3, and which can never be modified by the program.
Objects qualified const are placed into the program memory unless the
-mno-embedded-data
-mno-embedded-data
option is used.
6.10.2
Volatile Type Qualifier
The volatile type qualifier is used to tell the compiler that an object cannot be guar-
anteed to retain its value between successive accesses. This prevents the optimizer
from eliminating apparently redundant references to objects declared volatile
because it may alter the behavior of the program to do so.
anteed to retain its value between successive accesses. This prevents the optimizer
from eliminating apparently redundant references to objects declared volatile
because it may alter the behavior of the program to do so.
Any SFR which can be modified by hardware or which drives hardware is qualified as
volatile
volatile
, and any variables which may be modified by interrupt routines should use
this qualifier as well. For example:
extern volatile unsigned int WDTCON __attribute__((section("sfrs")));
The volatile qualifier does not guarantee that any access will be atomic, but the
compiler will try to implement this.
compiler will try to implement this.
The code produced by the compiler to access volatile objects may be different than
that to access ordinary variables, and typically the code will be longer and slower for
volatile
that to access ordinary variables, and typically the code will be longer and slower for
volatile
objects, so only use this qualifier if it is necessary. However failure to use
this qualifier when it is required may lead to code failure.
Another use of the volatile keyword is to prevent variables from being removed if
they are not used in the C/C++ source. If a non-volatile variable is never used, or
used in a way that has no effect on the program’s function, then it may be removed
before code is generated by the compiler.
they are not used in the C/C++ source. If a non-volatile variable is never used, or
used in a way that has no effect on the program’s function, then it may be removed
before code is generated by the compiler.