Agilent Technologies N5700 User Manual
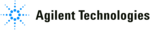
Output Programming Example
82
Series N5700 User’s Guide
Output Programming Example
This program sets the voltage, current, over-voltage, and the over-
current protection. When done, the program checks for instrument
errors and gives a message if there is an error.
current protection. When done, the program checks for instrument
errors and gives a message if there is an error.
Sub main_EZ()
Dim IDN As String
Dim IOaddress As String
Dim ErrString As String
' This variable controls the voltage
Dim VoltSetting As Double
' This variable measures the voltage
Dim MeasureVoltString As String
' This variable controls the current
Dim CurrSetting As Double
' This variable controls the over voltage protection setting
Dim overVoltSetting As Double
' This variable controls the over current protection
Dim overCurrentOn As Long
' These variables are necessary to initialize the VISA COM
Dim ioMgr As AgilentRMLib.SRMCls
Dim Instrument As VisaComLib.FormattedIO488
' The following line provides the VISA name of the GPIB interface
IOaddress = "GPIB0::5::INSTR"
' Use the following line instead for LAN communication
' IOaddress="TCPIP0::141.25.36.214"
' Use the following line instead for USB communication
' IOaddress = "USB0::2391::1799::US00000002"
' Initialize the VISA COM communication
Set ioMgr = New AgilentRMLib.SRMCls
Set Instrument = New VisaComLib.FormattedIO488
Set Instrument.IO = ioMgr.Open(GPIBaddress)
VoltSetting = 3 ' volts
CurrSetting = 1.5 ' amps
overVoltSetting = 10 ' volts
overCurrentOn = 1 ' 1 for on, 0 for off
With Instrument
' Send a power reset to the instrument
.WriteString "*RST"
' Query the instrument for the IDN string
.WriteString "*IDN?"
IDN = .ReadString
' Set the voltage
.WriteString "VOLT" & Str$(VoltSetting)